This Arduino Potentiometer circuit is a simple example that shows you how analog inputs work, and how you can use the Serial Monitor to learn about what is going on inside the chip.
In this quickstart guide, you’ll learn how to connect a potentiometer to an Arduino board and read out the voltage. This is a great practice circuit when you’re learning Arduino. The code is straightforward and the potentiometer connections are simple.
Parts Needed
- Arduino Uno
- Breadboard (and some breadboard wires)
- Potentiometer (Any value above 200 Ω will work)
Arduino Potentiometer Circuit
To connect a potentiometer to an Arduino, connect the middle pin of the potentiometer to an analog input pin on the Arduino. Then connect the outer pins to 5V and GND.
Connecting On a Breadboard
Here’s how you can connect a potentiometer to an Arduino by using a breadboard and some cables:
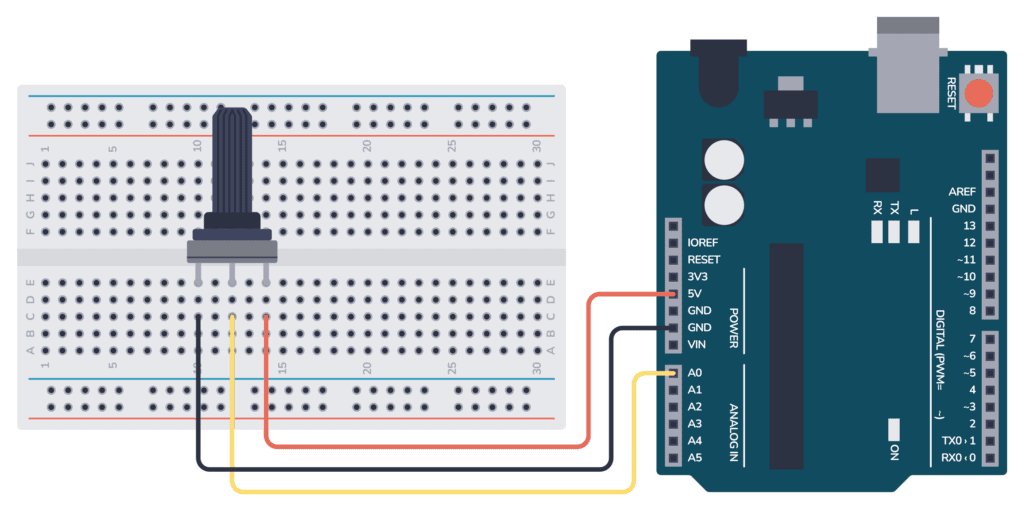
Arduino Potentiometer Code
This Arduino code is an example of reading the voltage from the potentiometer (connected to analog pin A0) and then printing the value of the analog reading to the Serial Monitor.
An analog pin will give you a value between 0 and 1023, where 0 means 0V and 1023 means the maximum voltage possible (usually 5V).
As with all Arduino code, you have the two main functions setup() and loop():
- Inside setup(), you need to configure the Serial port so that you can read out values.
- Inside loop(), you need to read the analog input and print this value out on the Serial port.
Check out the complete code:
void setup() {
// initialize serial communication at 9600 bits per second:
Serial.begin(9600);
}
void loop() {
// read the input on analog pin 0:
int sensorValue = analogRead(A0);
// print out the value you read in the Serial Montitor:
Serial.println(sensorValue);
delay(1); // delay in between reads for stability
}
How the Code Works
Inside the setup() function there is only one line: Serial.begin(9600);
This line sets up the serial port of the Arduino so that it’s possible to send data out from the Arduino and into your computer.
Inside the loop() function there are three lines:
int sensorValue = analogRead(A0);
: This line reads the analog voltage present at analog pin A0. It returns a value between 0 and 1023, representing the voltage level on the pin relative to the reference voltage (usually 5V for most Arduino boards). The value is stored in the variablesensorValue
.Serial.println(sensorValue);
: This line prints the value ofsensorValue
to the Serial Monitor so that you can read it.delay(100);
: This line adds a small delay of 100 milliseconds between each reading and printing. This delay prevents the code from running too quickly. This way it’s easier to read the readings on the Serial Monitor.
The end result of this code is that it continuously reads the analog voltage at pin A0, prints the reading (a number between 0 and 1023) to the Serial Monitor, and repeats the process in a loop.
When you turn the potentiometer, the voltage out from the potentiometer will change, and you can observe the changing values in the Serial Monitor. This is a helpful way to visualize the data and debug when things aren’t working as expected.
Using The Serial Monitor
To use the Serial Monitor to check the results from the code above, follow these steps:
- Connect your Arduino board to your computer using a USB cable.
- Upload the provided code to your Arduino board using the Arduino IDE.
- Open the Serial Monitor by clicking the magnifying glass icon or using the keyboard shortcut
Ctrl + Shift + M
(Windows/Linux) orCmd + Shift + M
(Mac). - Set the baud rate in the Serial Monitor to 9600 (or the same value as in the
Serial.begin()
function in the code). - Observe the continuous stream of numbers in the Serial Monitor. These numbers represent the analog readings from pin A0.
- Adjust the potentiometer to see how the readings change in real time.
- Close the Serial Monitor when you’re done by clicking the “x” button in the top-right corner.
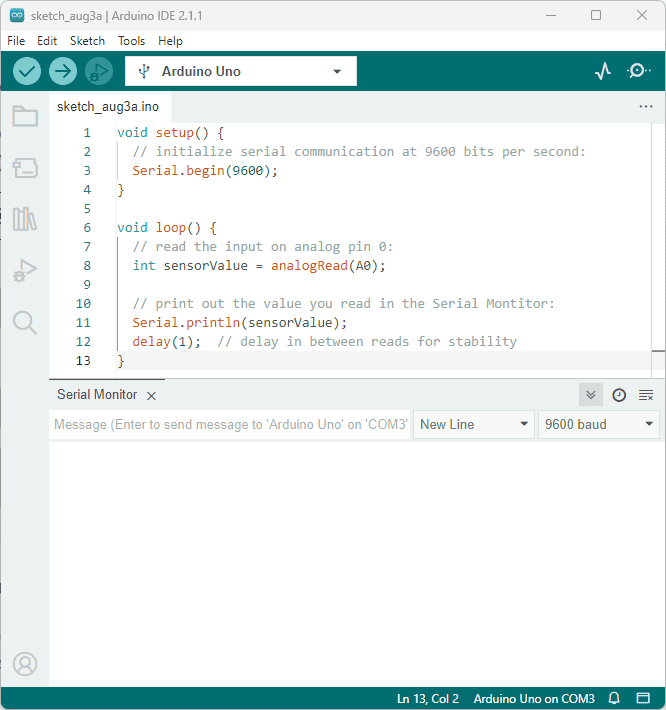
Copyright Build Electronic Circuits
No comments:
Post a Comment